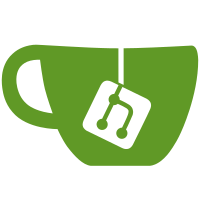
* Replace packet handler with templates * Move all packet functions into classes * Liberal use of Q_UNSUED * Add correct argument count to packets * Fix windows build issues * Partially implement argument type validation * Where applicable. * Checking if we can convert from a string to a string would be useless * Fix unit tests for AOPacket Co-authored-by: scatterflower <marisa@scatterflower.online> Co-authored-by: Salanto <62221668+Salanto@users.noreply.github.com>
37 lines
827 B
C++
37 lines
827 B
C++
#include "include/packet/packet_de.h"
|
|
#include "include/akashiutils.h"
|
|
#include "include/server.h"
|
|
|
|
#include <QDebug>
|
|
|
|
PacketDE::PacketDE(QStringList &contents) :
|
|
AOPacket(contents)
|
|
{
|
|
}
|
|
|
|
PacketInfo PacketDE::getPacketInfo() const
|
|
{
|
|
PacketInfo info{
|
|
.acl_permission = ACLRole::Permission::NONE,
|
|
.min_args = 1,
|
|
.header = "DE"};
|
|
return info;
|
|
}
|
|
|
|
void PacketDE::handlePacket(AreaData *area, AOClient &client) const
|
|
{
|
|
if (!client.checkEvidenceAccess(area))
|
|
return;
|
|
bool is_int = false;
|
|
int l_idx = m_content[0].toInt(&is_int);
|
|
if (is_int && l_idx < area->evidence().size() && l_idx >= 0) {
|
|
area->deleteEvidence(l_idx);
|
|
}
|
|
client.sendEvidenceList(area);
|
|
}
|
|
|
|
bool PacketDE::validatePacket() const
|
|
{
|
|
return AkashiUtils::checkArgType<int>(m_content.at(0));
|
|
}
|