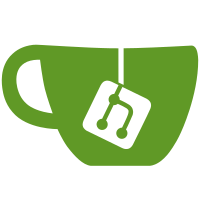
* Add clang-format * Multiple privatization changes "Participation handshake" this refers to the moment that the user's client sends the `askchaa` packet. * Server::m_clients is now private. Get a copy with Server::getClients() * Server::m_player_count is now private. Get a copy with Server::getPlayerCount() (Additional logic was added to handle the player count.) * AOClient::m_joined is now private. Get a copy with AOClient::hasJoined() * Added signal AOClient::joined(); will be emitted when the client first complete the participation handshake. * Renamed Server::updatePlayerCount to Server::playerCountUpdated * Privatized Server * Made Server members private: m_characters, m_areas, m_area_names * Added Server methods: getCharacters(), getAreas(), getAreaById(f_area_id), getAreaByName(f_area_name), getAreaNames(), getAreaName(f_area_id), getMusicList * Added Server helper methods: getCharacterCount(), getAreaCount() - This reduce code repetition of the following example: server->getCharacters().length(), server->getAreas().size() * Solved other merge conflicts * Added Server methods, various fixes * Added Server methods: getCharacterById(f_chr_id) * Various optimizations * More Server privatization changes * Made Server members private: db_manager, next_message_timer, can_send_ic_messages * Renamed Server members: * next_message_timer -> m_message_floodguard_timer * can_send_ic_message -> m_can_send_ic_message Added Server methods: getDatabaseManager, isMessageAllowed, startMessageFloodguard(f_duration) Made Server methods private: allowMessage * Added new fields to load for AreaData * Added fields: `area_message` (default: empty string) and `send_area_message_on_join` (default: false) * Added Server::clearAreaMessage * Cleaned up headers include (AOPacket excluded) * Removed most project file includes, moved to source file (cpp) * AOPacket was excluded because some methods modify the copy * Fix compile error when using MingW compiler * Appease clang by using proper or and putting it in parentheses * Remove extra semicolon
113 lines
3.8 KiB
C++
113 lines
3.8 KiB
C++
//////////////////////////////////////////////////////////////////////////////////////
|
|
// akashi - a server for Attorney Online 2 //
|
|
// Copyright (C) 2020 scatterflower //
|
|
// //
|
|
// This program is free software: you can redistribute it and/or modify //
|
|
// it under the terms of the GNU Affero General Public License as //
|
|
// published by the Free Software Foundation, either version 3 of the //
|
|
// License, or (at your option) any later version. //
|
|
// //
|
|
// This program is distributed in the hope that it will be useful, //
|
|
// but WITHOUT ANY WARRANTY; without even the implied warranty of //
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the //
|
|
// GNU Affero General Public License for more details. //
|
|
// //
|
|
// You should have received a copy of the GNU Affero General Public License //
|
|
// along with this program. If not, see <https://www.gnu.org/licenses/>. //
|
|
//////////////////////////////////////////////////////////////////////////////////////
|
|
#ifndef ADVERTISER_H
|
|
#define ADVERTISER_H
|
|
|
|
#include <QObject>
|
|
#include <QtNetwork>
|
|
|
|
/**
|
|
* @brief Represents the advertiser of the server. Sends current server information to masterserver.
|
|
*/
|
|
class Advertiser : public QObject
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
/**
|
|
* @brief Constructor for the HTTP_Advertiser class.
|
|
*/
|
|
explicit Advertiser();
|
|
|
|
/**
|
|
* @brief Deconstructor for the HTTP_Advertiser class. Yes, that's it. Can't say more about it.
|
|
*/
|
|
~Advertiser();
|
|
|
|
public slots:
|
|
|
|
/**
|
|
* @brief Establishes a connection with masterserver to register or update the listing on the masterserver.
|
|
*/
|
|
void msAdvertiseServer();
|
|
|
|
/**
|
|
* @brief Reads the information send as a reply for further error handling.
|
|
* @param reply Response data from the masterserver. Information contained is send to the console if debug is enabled.
|
|
*/
|
|
void msRequestFinished(QNetworkReply *f_reply);
|
|
|
|
/**
|
|
* @brief Updates the playercount of the server in the advertiser.
|
|
*/
|
|
void updatePlayerCount(int f_current_players);
|
|
|
|
/**
|
|
* @brief Updates advertisement values
|
|
*/
|
|
void updateAdvertiserSettings();
|
|
|
|
private:
|
|
/**
|
|
* @brief Pointer to the network manager, necessary to execute POST requests to the masterserver.
|
|
*/
|
|
QNetworkAccessManager *m_manager;
|
|
|
|
/**
|
|
* @brief Name of the server send to the masterserver. Changing this will change the display name in the serverlist
|
|
*/
|
|
QString m_name;
|
|
|
|
/**
|
|
* @brief Optional hostname of the server. Can either be an IP or a DNS name. Disabled automatic IP detection of ms3.
|
|
*/
|
|
QString m_hostname;
|
|
|
|
/**
|
|
* @brief Description of the server that is displayed in the client when the server is selected.
|
|
*/
|
|
QString m_description;
|
|
|
|
/**
|
|
* @brief Client port for the AO2-Client.
|
|
*/
|
|
int m_port;
|
|
|
|
/**
|
|
* @brief Websocket proxy port for WebAO users.
|
|
*/
|
|
int m_ws_port;
|
|
|
|
/**
|
|
* @brief Maximum amount of clients that can be connected to the server.
|
|
*/
|
|
int m_players;
|
|
|
|
/**
|
|
* @brief URL of the masterserver that m_manager posts to. This is almost never changed.
|
|
*/
|
|
QUrl m_masterserver;
|
|
|
|
/**
|
|
* @brief Controls if network replies are printed to console. Should only be true if issues communicating with masterserver appear.
|
|
*/
|
|
bool m_debug;
|
|
};
|
|
|
|
#endif // ADVERTISER_H
|