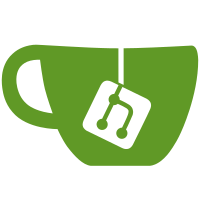
* Boilerplate structure for playerlist * Change id, character and area to private with get/set * WIP push * Restructured the project entirely * Implemented player list * Build against project-akashi.pro * Updated coverage location * Copy gcov files from the proper path * Update coverage to copy files * Coverage update. * Update main.yml * Disabled coverage for the time being * Reworked player list implementation, ... * Reworked player list implementation * No longer rely on JSON * Introduced moderation packets: ban, kick * A kick is a duration of 0 * A ban is a duration between -1 (permanent) and anything above 0 * Packet ZZ has been modified and now include a client id field for client-specific reports * Ban duration is now explicit. * Tweak to ban duration calculation * Resolve failing ZZ test --------- Co-authored-by: Salanto <62221668+Salanto@users.noreply.github.com>
42 lines
1.0 KiB
C++
42 lines
1.0 KiB
C++
#include "packet_pr.h"
|
|
|
|
PacketPR::PacketPR(QStringList &contents) :
|
|
AOPacket(contents)
|
|
{}
|
|
|
|
PacketPR::PacketPR(int f_id, UPDATE_TYPE f_update) :
|
|
AOPacket(QStringList{QString::number(f_id), QString::number(f_update)})
|
|
{}
|
|
|
|
PacketInfo PacketPR::getPacketInfo() const { return PacketInfo{.acl_permission = ACLRole::NONE, .min_args = 2, .header = "PR"}; }
|
|
|
|
void PacketPR::handlePacket(AreaData *area, AOClient &client) const
|
|
{
|
|
Q_UNUSED(area);
|
|
Q_UNUSED(client);
|
|
}
|
|
|
|
PacketPU::PacketPU(QStringList &contents) :
|
|
AOPacket(contents)
|
|
{}
|
|
|
|
PacketPU::PacketPU(int f_id, DATA_TYPE f_type, const QString &f_data) :
|
|
AOPacket(QStringList{QString::number(f_id), QString::number(f_type), f_data})
|
|
{}
|
|
|
|
PacketPU::PacketPU(int f_id, DATA_TYPE f_type, int f_data) :
|
|
PacketPU(f_id, f_type, QString::number(f_data))
|
|
{
|
|
}
|
|
|
|
PacketInfo PacketPU::getPacketInfo() const
|
|
{
|
|
return PacketInfo{.acl_permission = ACLRole::NONE, .min_args = 3, .header = "PU"};
|
|
}
|
|
|
|
void PacketPU::handlePacket(AreaData *area, AOClient &client) const
|
|
{
|
|
Q_UNUSED(area);
|
|
Q_UNUSED(client);
|
|
}
|